capsul.in_context module¶
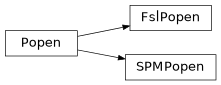
The in_context
module provides functions to call some external software from Capsul processes (SPM, FSL, etc.). The main functions perform calls to the software in a similar way as subprocess
functions (Popen
, call()
, check_call()
and subprocess.check_output()
). These functions are only valid when the software environment context is activated.
Activating the context is normally done using the with
statement on a CapsulEngine
object:
from capsul.engine import capsul_engine
from capsul.in_context.fsl import fsl_check_call
ce = capsul_engine()
# .. configure it ...
with ce:
fsl_check_call(['bet', '-h'])
capsul.in_context.fsl submodule¶
Specific subprocess-like functions to call FSL taking into account configuration stored in ExecutionContext. To functions and class in this module it is mandatory to activate an ExecutionContext (using a with statement). For instance:
from capsul.engine import capsul_engine
from capsul.in_context.fsl import fsl_check_call
ce = capsul_engine()
with ce:
fsl_check_call(['bet', '/somewhere/myimage.nii'])
For calling FSL command with this module, the first argument of command line must be the FSL executable without any path nor prefix. Prefix are used in Neurodebian install. For instance on Ubuntu 16.04 Neurodebian FSL commands are prefixed with “fsl5.0-“. The appropriate path and eventually prefix are added from the configuration of the ExecutionContext.
- class capsul.in_context.fsl.FslPopen(command, **kwargs)[source]¶
Equivalent to Python subprocess.Popen for FSL commands
Create new Popen instance.
- capsul.in_context.fsl.fsl_call(command, **kwargs)[source]¶
Equivalent to Python subprocess.call for FSL commands
- capsul.in_context.fsl.fsl_check_call(command, **kwargs)[source]¶
Equivalent to Python subprocess.check_call for FSL commands
- capsul.in_context.fsl.fsl_check_output(command, **kwargs)[source]¶
Equivalent to Python subprocess.check_output for FSL commands
- capsul.in_context.fsl.fsl_command_with_environment(command, use_prefix=True, use_runtime_env=True)[source]¶
Given an FSL command where first element is a command name without any path or prefix (e.g. “bet”). Returns the appropriate command to call taking into account the FSL configuration stored in the activated ExecutionContext.
Usinfg :func`fsl_env` is an alternative to this.
capsul.in_context.spm submodule¶
Specific subprocess-like functions to call SPM taking into account configuration stored in ExecutionContext. To functions and class in this module it is mandatory to activate an ExecutionContext (using a with statement). For instance:
from capsul.engine import capsul_engine
from capsul.in_context.spm import spm_check_call
ce = capsul_engine()
with ce:
spm_check_call(spm_batch_filename)
For calling SPM command with this module, the first argument of command line must be the SPM batch file to execute with Matlab.
- class capsul.in_context.spm.SPMPopen(spm_batch_filename, **kwargs)[source]¶
Equivalent to Python subprocess.Popen for SPM batch
Create new Popen instance.
- capsul.in_context.spm.spm_call(spm_batch_filename, **kwargs)[source]¶
Equivalent to Python subprocess.call for SPM batch